How to Create and Use Environment Variables in Python
Use environment variables to keep sensitive data out of your source code while maintaining a clean and flexible configuration setup.
Modern software development requires a secure and efficient way to manage configuration settings, especially sensitive information like API keys, database credentials, and authentication tokens. Hardcoding such details directly into your Python scripts poses significant security risks and reduces flexibility when deploying across different environments. This is where environment variables come in handy.
Environment variables allow you to separate configuration from code, making projects more maintainable and secure. By using a .env file alongside the python-dotenv library, you can easily load these variables into your Python application without exposing them in your source code. This approach is widely used in both local development and production environments to ensure security and scalability.
In this guide, we will break down the concept of environment variables and show you how to create a .env file, use it in Python projects, and follow best practices to keep your application secure and efficient.
What is a .env File?
Imagine you're working on a Python project and need to store some sensitive information—like an API key or database password. You SHOULD NOT write it directly in your Python code because doing so could expose it to others. Instead, you use a .env file to store such information securely. A .env file is simply a text file where you define key-value pairs, like this:
API_KEY=some_secret_key
DATABASE_URL=postgres://user:password@localhost:5432:mydb
How to Create and Use Environment Variables in Python
Step 1: Create a .env File in your project directory.
- Open your project folder.
- Create a new file and name it .env (yes, just .env, no extensions).
- Add your environment variables like this:
DATABASE_URL= some_database_connection
It is best practice for your variable to be stored in capital letters, and the name should be descriptive as to what the variable stores.
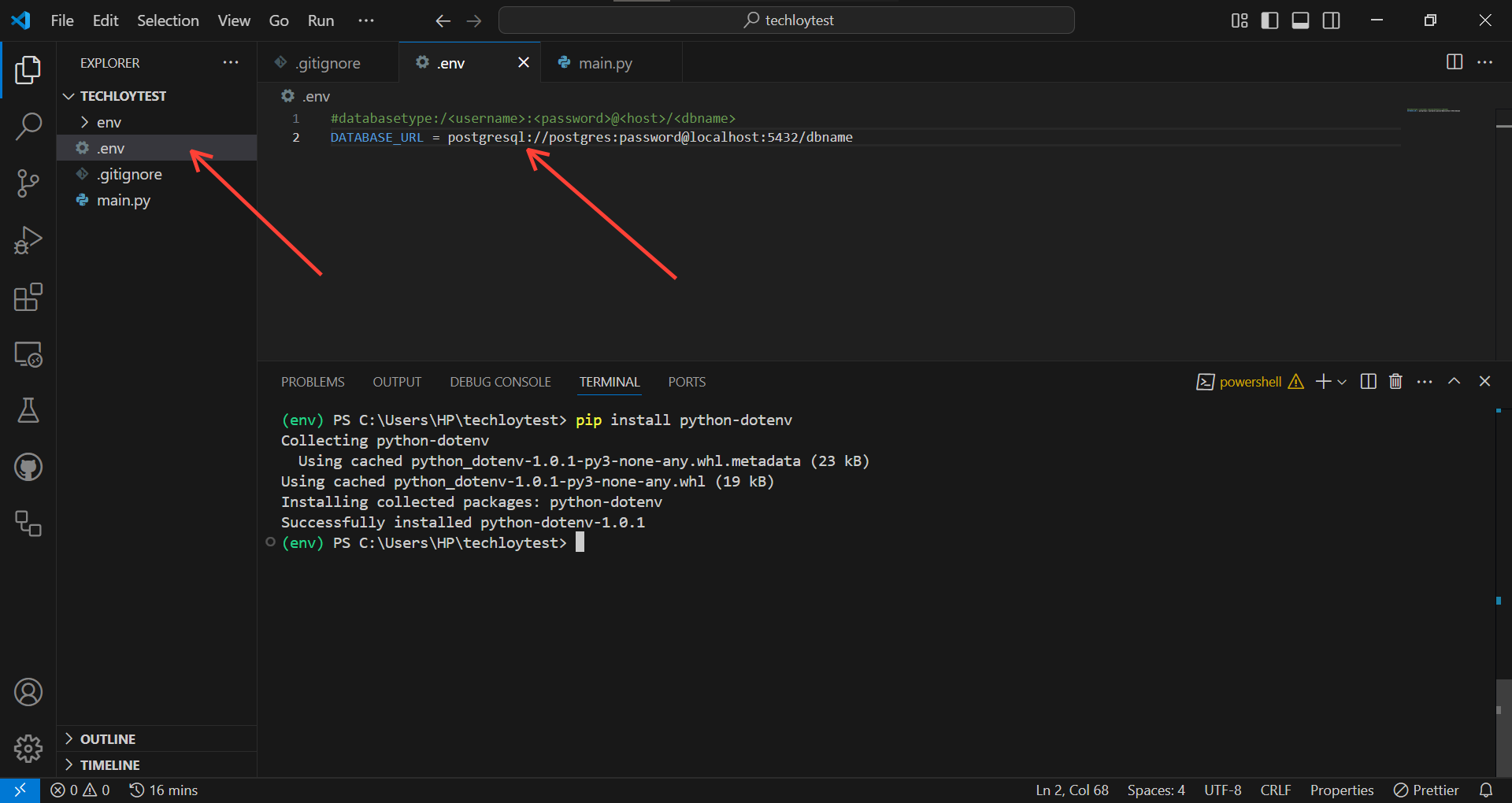
Step 2: Install python-dotenv
To read values from a .env file in Python, you need to install the python-dotenv package. Open your terminal and run:
pip install python-dotenv
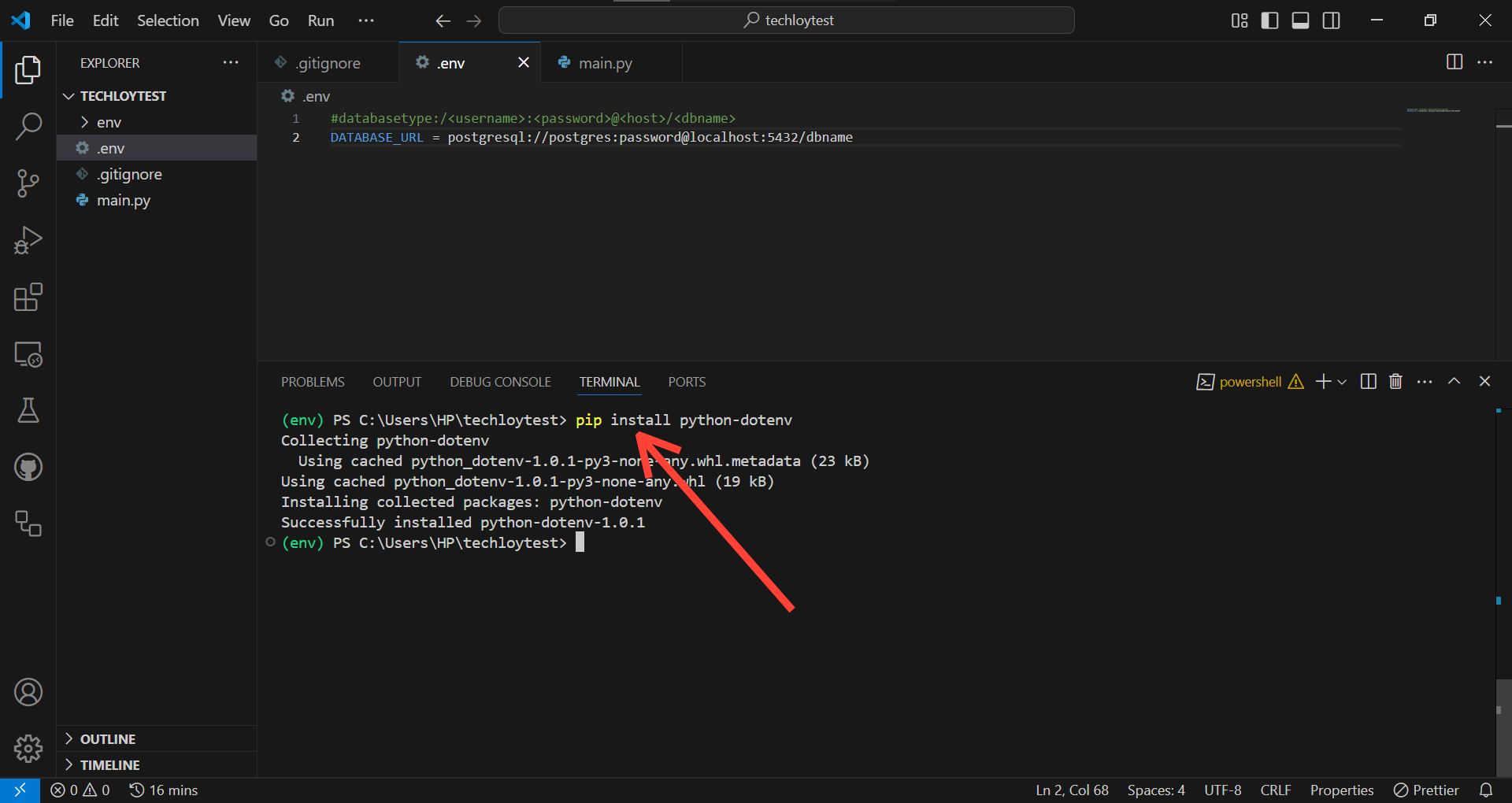
Step 3: Access the variables in the .env file
- In the Python code/file you want to use the variables stored in the .env file, first import "os".
import os
os is a built-in Python module, which provides functions for interacting with the operating system. In this case, we'll use it to access environment variables. - The next thing is to load the environment variables from the .env file using the load_dotenv() method. First import the load_env method from the dotenv package, then call the method.
from dotenv import load_dotenv
load_dotenv()
This method calls the load_dotenv function, which then looks for a .env file in the same directory as your Python script. If it finds one, it reads the key-value pairs from the file and sets them as environment variables. - After loading the environment variables, we can access them using the os.environ.get() method. In this code, we're accessing the DATABASE_URL environment variable like this:
DATABASE_URL = os.environ.get("DATABASE_URL")
os.environ.get() returns the value of the DATABASE_URL environment variable, which we then assign to the DATABASE_URL variable to be used anywhere in this file.
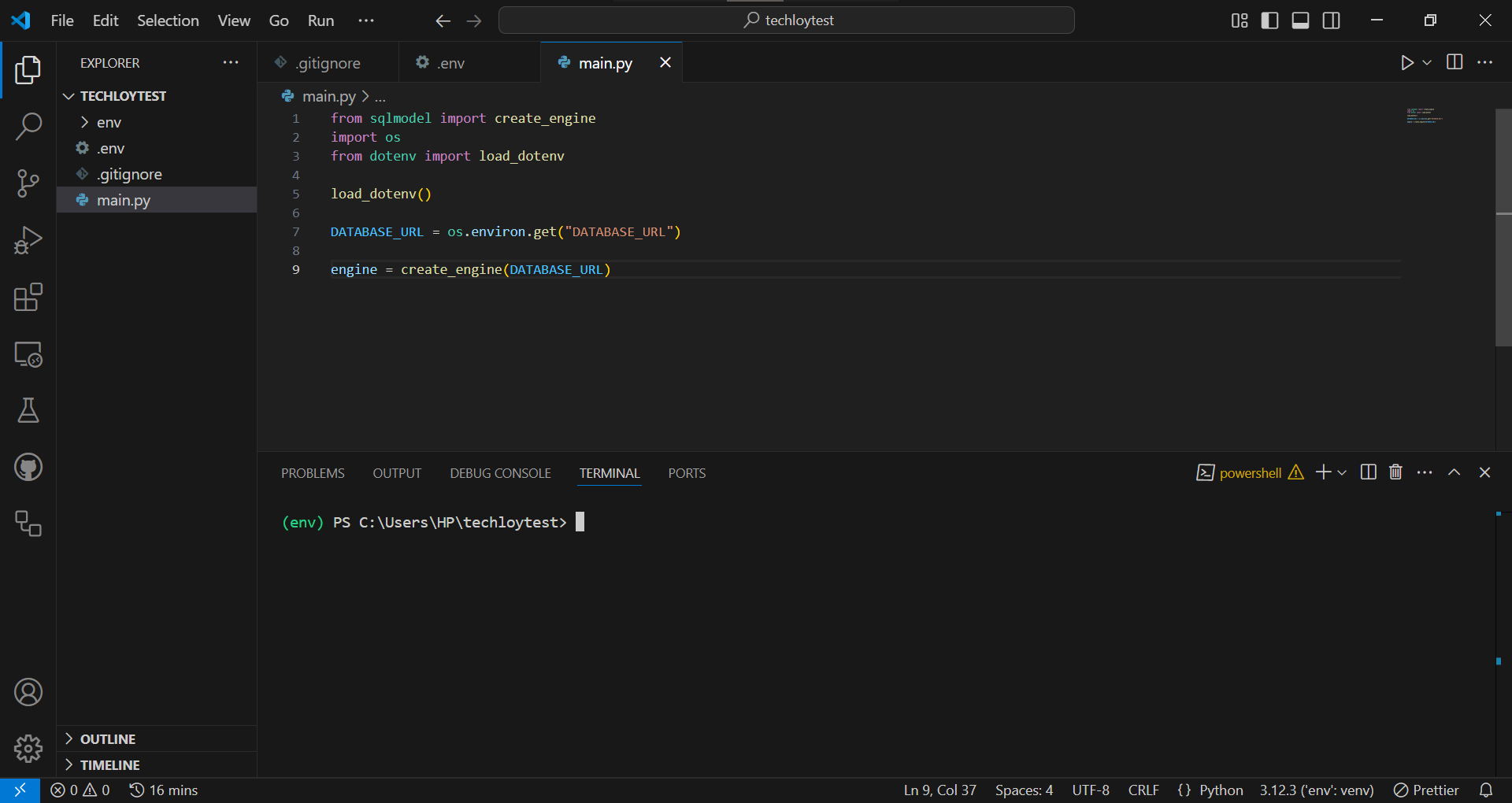
Best Practices for Using Environment Variables
- Avoid Hardcoding Sensitive Information – Store API keys and database credentials in .env files instead of writing them in your Python script.
- Use Descriptive Names – Name variables clearly (DATABASE_URL, API_SECRET_KEY, etc.).
- Use .env for Local Development – Keep configurations outside your codebase for better security and flexibility. Many cloud platforms, like Heroku and AWS, allow you to set environment variables without needing a .env file.
- Document Required Variables – Maintain a .env.example file (README.md) listing all the required variables without actual values. This helps team members or future developers understand what configuration is needed.
- Keep Your .env File Secure — Your .env file should never be shared in public repositories. To ensure it stays private, add it to your .gitignore file.
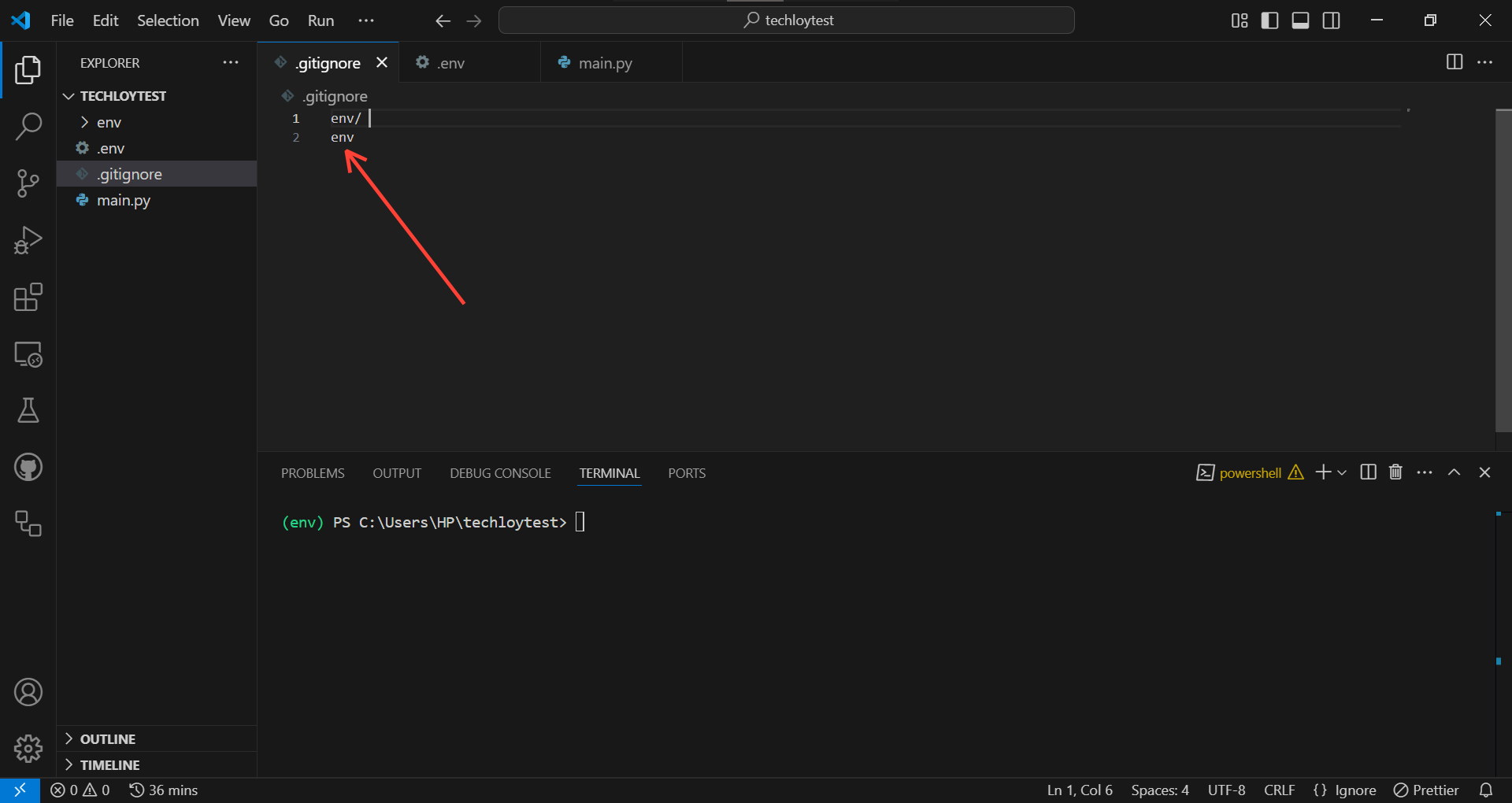
Conclusion
Using environment variables is essential for any Python developer looking to build secure and scalable applications. By using .env files and packages like python-dotenv you can keep sensitive data out of your source code while maintaining a clean and flexible configuration setup. This practice not only enhances security but also simplifies development and deployment.
So next time you're working on a Python project, make sure to utilize environment variables wisely. By following best practices and keeping your secrets safe, you’ll be on your way to writing more professional and secure applications
Image credit: Oyinebiladou Omemu/techloy.com